随便新建一个asp.net web项目,拖入即可。现在知道为什么游戏老是暴不出好装备,因为他的权重是非常小地。。。中午吃饭随机一下,想去哪一家,就把权重值设大一点,再中不了,就认命吧!
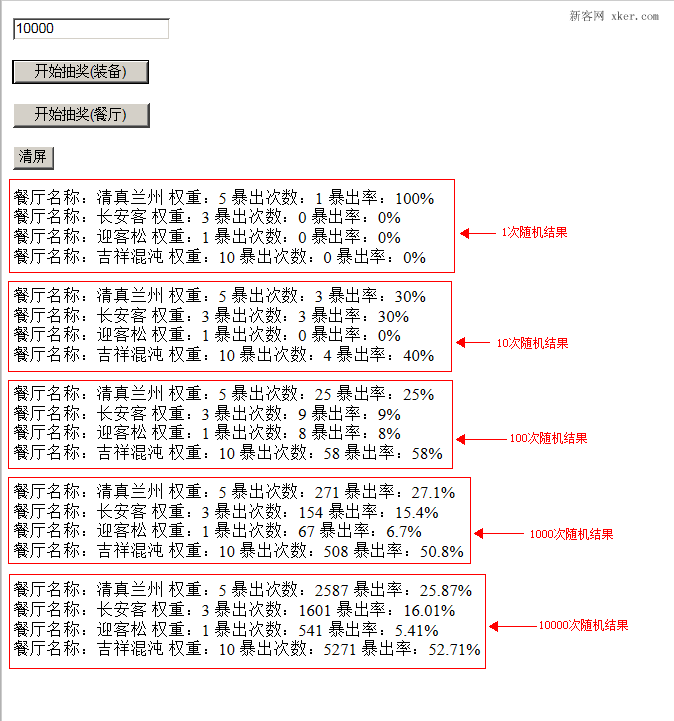
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>加权随机</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:TextBox ID="txtNum" runat="server" Text="100" ></asp:TextBox>
<br />
<br />
<asp:Button ID="btnRandom" runat="server" Text="开始抽奖(装备)" onclick="btnRandom_Click"/>
<br />
<br />
<asp:Button ID="btnRandomFood" runat="server" Text="开始抽奖(餐厅)" onclick="btnRandomFood_Click"/>
<br />
<br />
<asp:Button ID="btnClear" runat="server" Text="清屏" onclick="btnClear_Click"/>
<br />
<asp:Literal ID="lblResult" runat="server"></asp:Literal>
</div>
</form>
</body>
</html>
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
//<string,int>:<名称:权重>
public Dictionary<string, int> Goods = new Dictionary<string, int>();
public int TotalWeight = 0;
public class Good
{
/// <summary>
/// 名称
/// </summary>
public string Name
{
get;
set;
}
/// <summary>
/// 权重(大于等于1,否则出现的可能性为0)
/// </summary>
public int Weight
{
get;
set;
}
}
public List<Good> Result = new List<Good>();
protected void Page_Load(object sender, EventArgs e)
{
}
/// <summary>
/// 初始化装备 俺是《梦三国》玩家,嘿嘿
/// </summary>
protected void InitGoods()
{
Goods.Clear();
TotalWeight = 0;
Goods.Add("寒冰爪", 2); //神器(拳爪类)
Goods.Add("重毛皮", 300);
Goods.Add("小毛皮", 1000);
Goods.Add("轻毛皮", 1000);
Goods.Add("神之防具打造书", 5);
Goods.Add("传说武器打造书", 20);
Goods.Add("紫檀树枝", 300);
Goods.Add("硬毛皮", 600);
Goods.Add("勾魂水晶", 8);
Goods.Add("真龙炙舞剑", 30);
Goods.Add("董卓勾魂", 1); //神器中的神器(长柄类)
foreach (KeyValuePair<string, int> kvp in Goods)
{
TotalWeight += kvp.Value;
}
}
/// <summary>
/// 初始化餐厅,公司楼下餐厅比较多,只写几个,意思意思。。。
/// </summary>
protected void InitFood()
{
Goods.Clear();
TotalWeight = 0;
Goods.Add("清真兰州", 1);
Goods.Add("长安客", 1);
Goods.Add("迎客松", 1);
Goods.Add("吉祥混沌", 1);
foreach (KeyValuePair<string, int> kvp in Goods)
{
TotalWeight += kvp.Value;
}
}
protected int GetTryParse()
{
try
{
return int.Parse(txtNum.Text);
}
catch {
return 1;
}
}
//开始抽奖(装备)
protected void btnRandom_Click(object sender, EventArgs e)
{
InitGoods();
lblResult.Text = lblResult.Text + "<br/>";
int Count = GetTryParse();
for (int i = 1; i <= Count; i++)
{
Random rdm = new Random(GetRandomSeed());
int Weight = rdm.Next(1, TotalWeight + 1);
ProduceResult(Weight);
}
foreach (KeyValuePair<string, int> kvp in Goods)
{
int c = Result.Count(d => d.Name == kvp.Key);
double rate = c * 1.0 / Count * 1.0 * 100;
lblResult.Text = lblResult.Text + "物品名称:" + kvp.Key + " 权重:" + kvp.Value + " 暴出次数:" + c.ToString() + " 暴出率:" + rate + "%<br/>";
}
}
//开始抽奖(餐厅)
protected void btnRandomFood_Click(object sender, EventArgs e)
{
InitFood();
lblResult.Text = lblResult.Text + "<br/>";
int Count = GetTryParse();
for (int i = 1; i <= Count; i++)
{
Random rdm = new Random(GetRandomSeed());
int Weight = rdm.Next(1, TotalWeight + 1);
ProduceResult(Weight);
}
foreach (KeyValuePair<string, int> kvp in Goods)
{
int c = Result.Count(d => d.Name == kvp.Key);
double rate = c * 1.0 / Count * 1.0 * 100;
lblResult.Text = lblResult.Text + "餐厅名称:" + kvp.Key + " 权重:" + kvp.Value + " 暴出次数:" + c.ToString() + " 暴出率:" + rate + "%<br/>";
}
}
/// <summary>
/// 根据随机权重判断所在范围
/// </summary>
/// <param name="Weight"></param>
protected void ProduceResult(int Weight)
{
int min = 1;
int max = 1;
foreach (KeyValuePair<string, int> kvp in Goods)
{
max = min + kvp.Value - 1;
if (Weight >= min && Weight <= max)
{
Good g = new Good();
g.Name = kvp.Key;
g.Weight = kvp.Value;
Result.Add(g);
return;
}
min = max + 1;
}
}
/// <summary>
/// 随机种子值(防止速度过快造成的重复)
/// </summary>
/// <returns></returns>
private static int GetRandomSeed()
{
byte[] bytes = new byte[4];
System.Security.Cryptography.RNGCryptoServiceProvider rng = new System.Security.Cryptography.RNGCryptoServiceProvider();
rng.GetBytes(bytes);
return BitConverter.ToInt32(bytes, 0);
}
//清屏操作
protected void btnClear_Click(object sender, EventArgs e)
{
lblResult.Text = "";
}
}
以上所述就是本文的全部内容了,希望大家能够喜欢。
如果您喜欢本文请分享给您的好友,谢谢!